TestNG – Test Groups, Meta Group, Default
Group
Grouping test methods is one of the most important features of TestNG. In TestNG
users can group multiple test methods into a named group. You can also execute
a particular set of test methods belonging to a group or multiple groups. This
feature allows the test methods to be segregated into different sections or
modules. For example, you can have a set of tests that belong to sanity test
where as others may belong to regression tests. You can also segregate the tests based on the functionalities/features that the test method verifies. This
helps in executing only a particular set of tests as and when required.
Example:
Group Test
Let’s create a test class as GroupTestExample.java, which contains certain test methods that belong to a group.
package
Grouping;
import
org.testng.annotations.Test;
public class
GroupTestExample {
@Test(groups
= { "test-group" })
public void
testMethodOne() {
System.out.println("Test
method one belonging to group.");
}
@Test
public void
testMethodTwo() {
System.out.println("Test
method two not belonging to group.");
}
@Test(groups
= { "test-group" })
public void
testMethodThree() {
System.out.println("Test
method three belonging to group.");
}
}
If you will run above test in eclipse normally,
then test execution does not consider the group for execution and hence
executes all the tests in the specified test class.
If you want to execute methods under a certain
group only, then you will execute them in either one manner discussed in
following two sections.
1.
Running a TestNG group through Eclipse
In the earlier
section we created a test class with certain test methods that belonged to a
test group. Now let’s run the group of tests using eclipse.
1) Go to Run | Run
Configurations
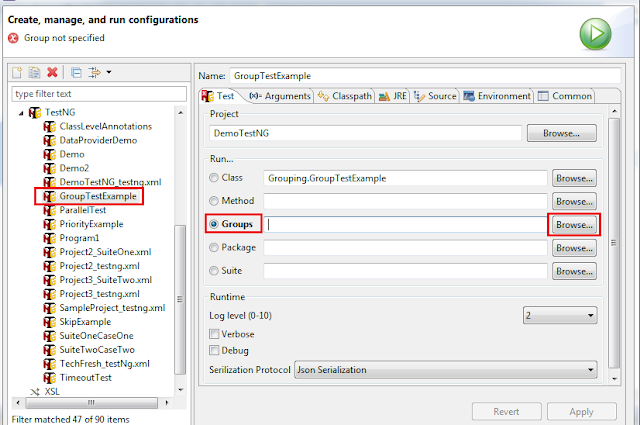
2. Select your TestNG Class from the list
of available
Go to the Groups
section and click on the Browse button. It will open a new popup window as
shown in below.
Select the group
which you would like to execute from the list, in this case it is test-group.
Click on the Apply
button and then click on Run. The
following results will be shown in the TestNG’s Results window of Eclipse:
[TestNG] Running:
C:\Users\Sujoy\AppData\Local\Temp\testng-eclipse--1770406877\testng-customsuite.xml
Test method one
belonging to group.
Test method three
belonging to group.
[Utils] Attempting to
create D:\WorkSpace\DemoTestNG\test-output\DemoTestNG by
groups\GRP-test-group.xml
[Utils] Directory
D:\WorkSpace\DemoTestNG\test-output\DemoTestNG by groups exists: true
PASSED: testMethodOne
PASSED: testMethodThree
===============================================
GRP-test-group
Tests run: 2, Failures: 0, Skips: 0
===============================================
Congratulations, you have successfully executed
test methods that belonged to a particular group using the TestNG runner
configuration in Eclipse.
Running a TestNG group through testng.xml
Configure your testng.xml file as below.
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Time test Suite" verbose="1">
<test name="Group Test">
<groups>
<run>
<include name="test-group" />
</run>
</groups>
<classes>
<class name="Grouping.GroupTestExample" />
</classes>
</test>
</suite>
This xml file contains only one test inside a
suite. This contains the groups section defined by using the groups tag as
shown in the code.
The run
tag represents the group that needs to be run.
The include
tag represents the name of the group that needs to be executed.
Once executed the above xml file you will see the
following result.
[TestNG] Running:
D:\WorkSpace\DemoTestNG\testng.xml
Test method one
belonging to group.
Test method three
belonging to group.
===============================================
Time test Suite
Total tests run: 2,
Failures: 0, Skips: 0
===============================================
Congratulations, you have successfully executed
test methods that belonged to a particular group using testng.xml file.
Writing
tests which belong to multiple groups
Earlier we learned about creating tests that
belonged to a single group, but TestNG allows test methods
to belong to multiple groups also. This can be done by
providing the group names as an array in the groups attribute of the
@Test
annotation.
Create a sample class as below.
package
Grouping;
import
org.testng.annotations.Test;
public class
MultiGroupExample {
@Test(groups = { "group-one" })
public void
testMethodOne() {
System.out.println("Test
method one belonging to group.");
}
@Test(groups
= { "group-one", "group-two" })
public void
testMethodTwo() {
System.out.println("Test
method two belonging to both group.");
}
@Test(groups
= { "group-two" })
public void
testMethodThree() {
System.out.println("Test
method three belonging to group.");
}
}
The above class contains three test methods. Two
of the test methods belong to one group each, where as one of the methods
belongs to two groups, group-one and group-two respectively.
Now
Configure your testng.xml file as below.
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Multi Group Suite" verbose="1">
<test name="Group Test one">
<groups>
<run>
<include name="group-one" />
</run>
</groups>
<classes>
<class name="Grouping.MultiGroupExample" />
</classes>
</test>
<test name="Group Test two">
<groups>
<run>
<include name="group-two" />
</run>
</groups>
<classes>
<class name="Grouping.MultiGroupExample" />
</classes>
</test>
</suite>
Once
executed the above testng.xml file, You
will see the following test results:
[TestNG] Running:
D:\WorkSpace\DemoTestNG\testng.xml
Test method one
belonging to group.
Test method two
belonging to both group.
Test method three
belonging to group.
Test method two
belonging to both group.
===============================================
Multi Group Suite
Total tests run: 4,
Failures: 0, Skips: 0
===============================================
As you can see in the test result,
testMethodTwo()
is executed in both the tests of the test suite. This is
because it belongs to both of the groups whose test methods are executed by
TestNG.
Including and excluding groups
TestNG also
allows you to include and
exclude certain groups from test execution. This helps in
executing only a particular set of tests and excluding certain tests.
Create a sample program as below:
package
Grouping;
import
org.testng.annotations.Test;
public class
IncludeExcludeGroup {
@Test(groups
= { "include-group" })
public void
testMethodOne() {
System.out.println("Test
method one belonging to include group.");
}
@Test(groups
= { "include-group" })
public void
testMethodTwo() {
System.out.println("Test
method two belonging to include group.");
}
@Test(groups
= { "include-group", "exclude-group" })
public void
testMethodThree() {
System.out.println("Test
method three belonging to exclude/include groups.");
}
}
The above class contains three test methods that
print a message onto console when executed. All the three methods belong to a
group include-group whereas the
testMethodThree()
method belongs to both include-group and exclude-group.
Now
Configure your testng.xml file as below.
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="IncludeExclude Group Suite" verbose="1">
<test name="IncludeExclude Group Test">
<groups>
<run>
<include name="include-group" />
<exclude name="exclude-group" />
</run>
</groups>
<classes>
<class name="Grouping.IncludeExcludeGroup" />
</classes>
</test>
</suite>
Once
executed the above testng.xml file, You
will see the following test results:
[TestNG] Running:
D:\WorkSpace\DemoTestNG\testng.xml
Test method one
belonging to include group.
Test method two
belonging to include group.
===============================================
IncludeExclude Group
Suite
Total tests run: 2,
Failures: 0, Skips: 0
===============================================
Using regular expressions
While
configuring your tests for including or excluding groups, TestNG allows the
user to use regular
expressions. This is similar to including and excluding the
test methods that we covered earlier. This helps users to include and exclude
groups based on a name search.
Create a sample program as below:
package
Grouping;
import
org.testng.annotations.Test;
public class
RegularExpressionGroupTest {
@Test(groups = { "include-test-one" })
public void
testMethodOne() {
System.out.println("===Test
method one===");
}
@Test(groups
= { "include-test-two" })
public void
testMethodTwo() {
System.out.println("===Test
method two===");
}
@Test(groups
= { "test-one-exclude" })
public void
testMethodThree() {
System.out.println("===Test
method three===");
}
@Test(groups
= { "test-two-exclude" })
public void
testMethodFour() {
System.out.println("===Test
method Four===");
}
}
Now
Configure your testng.xml file as below.
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Regular Exp. Group Suite" verbose="1">
<test name="Regular Exp. Test">
<groups>
<run>
<include name="include.*" />
<exclude name=".*exclude" />
</run>
</groups>
<classes>
<class name="Grouping.RegularExpressionGroupTest"
/>
</classes>
</test>
</suite>
The above testng.xml contains a simple test in
which all the groups with a name starting with include are included, whereas all the groups with name ending with exclude are excluded from your test
execution.
Once
executed the above testng.xml file, You will see
the following test results:
[TestNG] Running:
D:\WorkSpace\DemoTestNG\testng.xml
===Test method one===
===Test method two===
===============================================
Regular Exp. Group
Suite
Total tests run: 2,
Failures: 0, Skips: 0
===============================================
Here TestNG executed two methods that belong to
groups with a name starting with include and excluded the test methods that
belonged to groups with names ending with exclude.
Note: To use regular expressions to
include and exclude groups you have to use .* for matching names. We can also
use it for searching groups that contains a certain string in their names by
using the expression at start and end of the search string (for example, .*name.*).
Assigning
Default group
Sometimes we may need to assign a default group to a set of test methods that belong
to a class. This way all the public methods that belong to the particular class
will automatically become TestNG test methods and become part of the said
group.
This can be achieved by using the
@Test
annotation at class level and defining the default group in the
said @Test
annotation.
Example:
package
Grouping;
import
org.testng.annotations.Test;
@Test(groups={"default-group"})
public class
DefaultGroup {
public void
testMethodOne(){
System.out.println("Test
method one.");
}
public void
testMethodTwo(){
System.out.println("Test
method two.");
}
@Test(groups={"test-group"})
public void
testMethodThree(){
System.out.println("Test
method three.");
}
}
Group
of groups or ‘MetaGroups’
TestNG allows users to create groups out of
existing groups and then use them during the creation of the test suite.
You can create
new groups by including and excluding certain groups and then use them.
Create a sample program as below:
package
Grouping;
import org.testng.annotations.Test;
public class MetaGroups {
@Test(groups = { "include-test-one" })
public void
testMethodOne() {
System.out.println("Test
method one");
}
@Test(groups
= { "include-test-two" })
public void testMethodTwo()
{
System.out.println("Test
method two");
}
@Test(groups
= { "test-one-exclude" })
public void
testMethodThree() {
System.out.println("Test
method three");
}
@Test(groups
= { "test-two-exclude" })
public void
testMethodFour() {
System.out.println("Test
method Four");
}
}
Now
Configure your testng.xml file as below.
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Group of group Suite" verbose="1">
<test name="Group of group Test">
<groups>
<define name="include-group">
<include name="include-test-one" />
<include name="include-test-two" />
</define>
<define name="exclude-group">
<include name="test-one-exclude" />
<include name="test-two-exclude" />
</define>
<run>
<include name="include-group" />
<exclude name="exclude-group" />
</run>
</groups>
<classes>
<class name="Grouping.MetaGroups" />
</classes>
</test>
</suite>
Here two groups of groups have been defined inside
the test, and then these groups are used for test execution. The MetaGroup is created using the define
tag inside the groups tag. The name of the new group is
defined using the name attribute under the define tag. Groups are included and
excluded from the new group by using the
include and exclude tags.
Once
executed the above testng.xml file, You will see
the following test results:
[TestNG] Running:
D:\WorkSpace\DemoTestNG\testng.xml
Test method one
Test method two
===============================================
Group of group Suite
Total tests run: 2,
Failures: 0, Skips: 0
===============================================
Here, testNG executed only two methods, as
mentioned in the included-group group and excluded the test methods that belong
to excluded-group. You can define as many groups of groups as you want.
That’s all related to test groups in TestNG.
No comments:
Post a Comment